第121回【Python】デフォルト引数
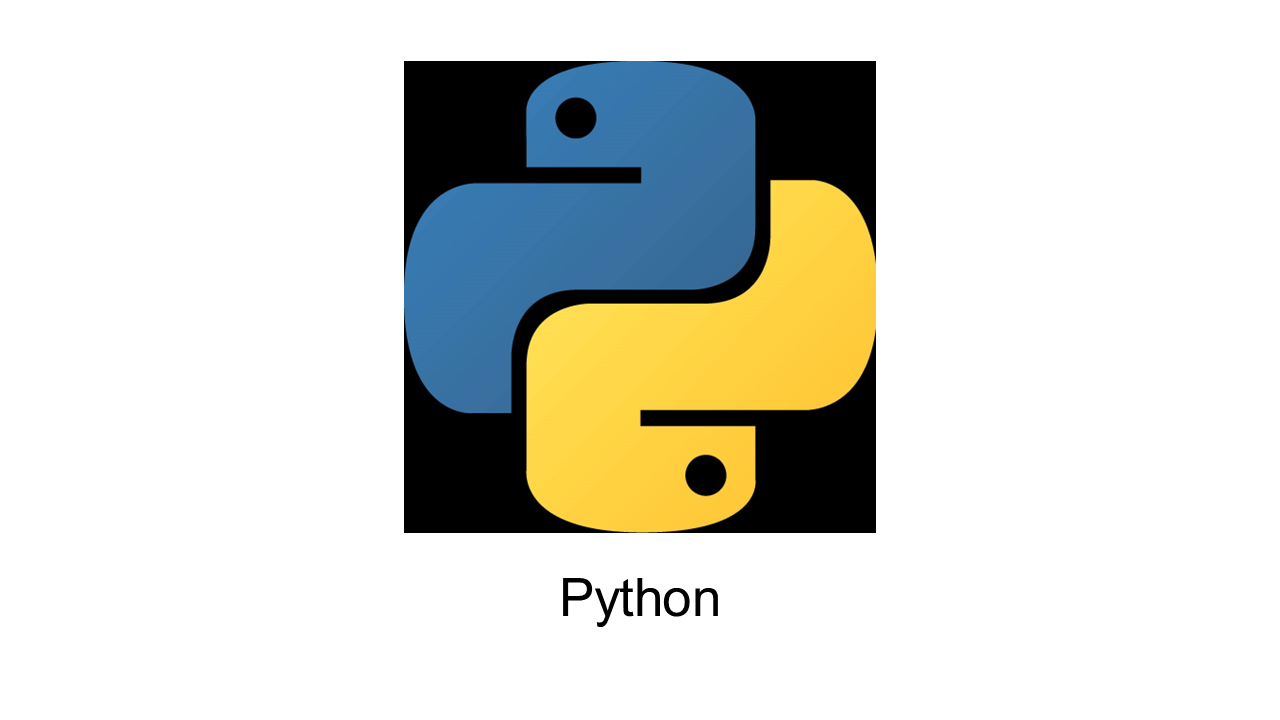
現在取り組んでいるのは、paiza ラーニング問題集「クラス・構造体メニュー」になります。
はじめに
猫とキャンプと野球観戦と AWS が大好きな旦那、LeoSaki です。モフモフしたい。
Python をゼロから勉強してみよう、のコーナー 121 回目です。
たまに PHP を書くことがあります。以前は結構書いていたはずなのですが、忘れているものです。いろいろ調べながらでないとうまくいかず、時間を無駄にしてしまいます。あとよくやるのが、閉じカッコや締めを忘れてしまうやつ。Python 使う人あるあるじゃないかなぁ。
それでは、今日も頑張ってみようと思います。
デフォルト引数
居酒屋で働きながらクラスの勉強をしていたあなたは、お客さんをクラスに見立てることで店内の情報を管理できることに気付きました。
全てのお客さんは、ソフトドリンクと食事を頼むことができます。加えて 20 歳以上のお客さんはお酒を頼むことができます。
20 歳未満のお客さんがお酒を頼もうとした場合はその注文は取り消されます。
また、お酒(ビールを含む)を頼んだ場合、以降の全ての食事の注文 が毎回 200 円引きになります。
今回、この居酒屋でビールフェスをやることになり、ビールの注文が相次いだため、いちいちビールの値段である 500 円を書くのをやめ、伝票に注文の種類と値段を書く代わりに 0 とだけを書くことになりました。
店内の全てのお客さんの数と注文の回数、各注文をしたお客さんの番号とその内容が与えられるので、各お客さんの会計を求めてください。
N K
a_1
...
a_N
n_1 o_1
...
n_K o_K
・ 1 行目では、お客さんの人数 N と注文の回数 K が与えられます。
・ 続く N 行のうち i 行目(1 ≦ i ≦ N)では、i 番目のお客さんの年齢が与えられます。
・ 続く K 行では、頼んだお客さんの番号 n_i , 注文を表す文字列 o_i が与えられます。
・ o_i では、注文の種類 s_i と 値段 m_i (1 ≦ i ≦ K) を表す文字列 “s_i m_i" または、ビールの注文を表す “0" が与えられます。
すべてのテストケースにおいて、以下の条件をみたします。
・ 1 ≦ N , K ≦ 1000
・ 1 ≦ a_i ≦ 100 (1 ≦ i ≦ N)
・ 1 ≦ n_i ≦ N (1 ≦ i ≦ K)
o_i (1 ≦ i ≦ K) は次のうちのいずれかの形式です。
・ “s_i m_i"
1 ≦ s_i ≦ N (1 ≦ i ≦ K) は “food" , “softdrink" , “alcohol" のいずれかです。
food , softdrink , alcohol はその注文が食事・ソフトドリンク・お酒であることを表しています。また、300 ≦ m_i ≦ 5000 です。
・ “0"
その注文がビールであることを表す。
入力例
3 5
19
43
22
2 0
2 food 4333
1 0
2 0
1 food 4606
出力例
4606
5133
0
ビール 1 杯 500 円はなかなか微妙なところをついてくる。
Python
class Underage:
def __init__(self):
self.total = 0
def alcohol(self,price=500):
pass
def softdrink(self,price):
self.total += price
def food(self,price):
self.total += price
def total_price(self):
return self.total
class Overage(Underage):
def __init__(self):
super().__init__()
self.flg = False
def alcohol(self,price=500):
self.flg = True
self.total += price
def food(self,price):
if self.flg:
self.total += price - 200
else:
self.total += price
N,K = map(int,input().split())
guests = [None] * N
for i in range(N):
a = int(input())
if a < 20:
guests[i] = Underage()
else:
guests[i] = Overage()
for i in range(K):
S = input().split()
idx = int(S[0]) - 1
order = S[1]
if order == '0':
guests[idx].alcohol()
else:
price = int(S[2])
if order == 'food':
guests[idx].food(price)
elif order == 'alcohol':
guests[idx].alcohol(price)
elif order == 'softdrink':
guests[idx].softdrink(price)
for guest in guests:
print(guest.total_price())
VBA
## Underage
Private total As Long
Private Sub Class_Initialize()
total = 0
End Sub
Property Let alcohol(price)
End Property
Property Let softdrink(price)
total = total + price
End Property
Property Let food(price)
total = total + price
End Property
Property Get totalPrice()
totalPrice = total
End Property
## Overage
Implements Underage
Private total As Long
Private flg As Boolean
Private Sub Class_Initialize()
total = 0
flg = False
End Sub
Property Let Underage_food(price)
If flg Then
total = total + price - 200
Else
total = total + price
End If
End Property
Property Let Underage_softdrink(price)
total = total + price
End Property
Property Let Underage_alcohol(price)
flg = True
If price = 0 Then
total = total + 500
Else
total = total + price
End If
End Property
Property Get Underage_totalPrice()
Underage_totalPrice = total
End Property
## 標準モジュール
Sub class_primer__set_default()
NK = Split(Cells(1, 1), " ")
N = Val(NK(0))
K = Val(NK(1))
Dim cls() As New Underage
ReDim cls(N - 1)
For i = 0 To N - 1
age = Cells(i + 2, 1)
If age < 20 Then
Set cls(i) = New Underage
Else
Set cls(i) = New Overage
End If
Next
For i = 0 To K - 1
S = Split(Cells(i + N + 2, 1), " ")
idx = Val(S(0)) - 1
ord = S(1)
If ord = "0" Then
cls(idx).alcohol = 0
Else
price = Val(S(2))
If ord = "food" Then
cls(idx).food = price
ElseIf ord = "alcohol" Then
cls(idx).alcohol = price
ElseIf ord = "softdrink" Then
cls(idx).softdrink = price
End If
End If
Next
For i = 0 To N - 1
Debug.Print cls(i).totalPrice()
Next
End Sub
最後に
うーん・・・。VBA では、引数に optional を付けてデフォルト値を設定できる、はずなんですが、Property ではダメなんでしょうか。デフォルト値の設定が叶わず、ありきたりのやり方に落ち着いてしまいました。
Property をやめて、Function にでもしようかとも思いましたが、問題に副えばそうした方がいいのでしょうけれど、Class の使い方としてあっているのかどうか悩むところ。解決策を知っている方がいらっしゃれば教えてください。
引き続き、よろしくお願いいたします!
ディスカッション
コメント一覧
まだ、コメントがありません